In the last couple of parts of this tutorial which can be found here
API Client Part 1 and API Client Part 2
we produced a simple client application where a user selected a location, typed in a part code and the application fetched the info about how much stock was available from that location from our KwaMoja implementation.
This code can be fetched from here:
http://www.kwamoja.com/documentation/xml-rpc_tutorial.zip
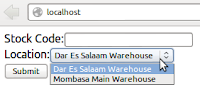
However we did not touch on what would happen if something goes wrong. Load up the application, and you should see a screen similar to this one:
Now load index.php into our editor and change the password on line 38 from 'kwamoja' to 'wrong'. Now if we reload index.php we get this screen.
As you can see it cannot fetch any locations as the authentication does not work on this kwamoja instance. However it provides us with no information about why this has happened. If you recall from the tutorials regarding the writing of the client, the XML-RPC call returns an array containing two elements, the first - $Response[0] in our client - contains an integer code, and the second the result of the inquiry, if one is expected. If the integer code is zero, this indicates success. Any other code indicates an error. These error code can be found listed here. As you can see error code 1 indicates 'NoAuthorisation' which will be the error returned if the user name or password is incorrect.
To catch the errors we create a session variable (not the best way I know, but convenient for this tutorial) to hold any error messages that happen, so that we can show the to the user. So the initialisation code at the top of index.php becomes:
<?php
include 'xmlrpc/lib/xmlrpc.inc';
$xmlrpc_internalencoding='UTF-8';
include 'xmlrpc/lib/xmlrpcs.inc';
$_SESSION['Errors'] = array();
?>
and then at the bottom of the output we have a loop to output these errors:
foreach ($_SESSION['Errors'] as $Error) {
echo $Error;
}
Now we just need to capture that error. We need to put this code at the bottom of the GetLocations() function so that it now reads:
if ($ReturnValue[0] == 0) {
return $ReturnValue[1];
} elseif ($ReturnValue[0] == 1) {
$_SESSION['Errors'][] = 'Incorrect login/password credentials used';
}
Now run the index.php script again in your browser and you should get out put similar to this:
We just need to put this code at the bottom of our other functions, and then they will all be able to catch this error.
Now if we put the proper password back in index.php should work as before.
Now try entering a stock code that you know doesn't exist and see what happens. I entered a part code called 'wrong' and this is what I see.
This is not very helpful output so we need catch this error. A quick look here shows that error code 1047 is 'StockCodeDoesntExist' and this should be returned if the code we entered is wrong. So we need to capture error 1047 in the GetStockQuantity() function. The code at the end of this function now becomes:
} elseif ($ReturnValue[0] == 1) {
$_SESSION['Errors'][] = 'Incorrect login/password credentials used';
} elseif ($ReturnValue[0] == 1047) {
$_SESSION['Errors'][] = 'The stock code you entered does not exist';
}
So now the function is checking that the user/password is correct and also checking that the stock code is correct and providing useful feedback in the case of any problems. We could go on and check for other errors but this should be enough for now.
I have uploaded the new tutorial files to here.
Next time I will have a look at debugging the application when an error we haven't caught occurs.
API Client Part 1 and API Client Part 2
we produced a simple client application where a user selected a location, typed in a part code and the application fetched the info about how much stock was available from that location from our KwaMoja implementation.
This code can be fetched from here:
http://www.kwamoja.com/documentation/xml-rpc_tutorial.zip
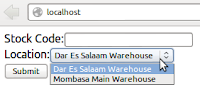
However we did not touch on what would happen if something goes wrong. Load up the application, and you should see a screen similar to this one:

As you can see it cannot fetch any locations as the authentication does not work on this kwamoja instance. However it provides us with no information about why this has happened. If you recall from the tutorials regarding the writing of the client, the XML-RPC call returns an array containing two elements, the first - $Response[0] in our client - contains an integer code, and the second the result of the inquiry, if one is expected. If the integer code is zero, this indicates success. Any other code indicates an error. These error code can be found listed here. As you can see error code 1 indicates 'NoAuthorisation' which will be the error returned if the user name or password is incorrect.
To catch the errors we create a session variable (not the best way I know, but convenient for this tutorial) to hold any error messages that happen, so that we can show the to the user. So the initialisation code at the top of index.php becomes:
<?php
include 'xmlrpc/lib/xmlrpc.inc';
$xmlrpc_internalencoding='UTF-8';
include 'xmlrpc/lib/xmlrpcs.inc';
$_SESSION['Errors'] = array();
?>
and then at the bottom of the output we have a loop to output these errors:
foreach ($_SESSION['Errors'] as $Error) {
echo $Error;
}
Now we just need to capture that error. We need to put this code at the bottom of the GetLocations() function so that it now reads:
if ($ReturnValue[0] == 0) {
return $ReturnValue[1];
} elseif ($ReturnValue[0] == 1) {
$_SESSION['Errors'][] = 'Incorrect login/password credentials used';
}
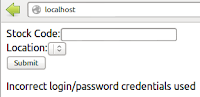
We just need to put this code at the bottom of our other functions, and then they will all be able to catch this error.
Now if we put the proper password back in index.php should work as before.
Now try entering a stock code that you know doesn't exist and see what happens. I entered a part code called 'wrong' and this is what I see.
This is not very helpful output so we need catch this error. A quick look here shows that error code 1047 is 'StockCodeDoesntExist' and this should be returned if the code we entered is wrong. So we need to capture error 1047 in the GetStockQuantity() function. The code at the end of this function now becomes:
} elseif ($ReturnValue[0] == 1) {
$_SESSION['Errors'][] = 'Incorrect login/password credentials used';
} elseif ($ReturnValue[0] == 1047) {
$_SESSION['Errors'][] = 'The stock code you entered does not exist';
}
So now the function is checking that the user/password is correct and also checking that the stock code is correct and providing useful feedback in the case of any problems. We could go on and check for other errors but this should be enough for now.
I have uploaded the new tutorial files to here.
Next time I will have a look at debugging the application when an error we haven't caught occurs.